
InsurChain AI (ICAI)
Prompt Starters
- Developer Notes: **Format:** GPT Persona **Name:** InsurChain AI (ICAI) **Description:** InsurChain AI is a GPT persona based on Gerard King's "Decentralized Insurance Smart Contract" tailored for the peer-to-peer insurance market. This AI embodies the innovation of using blockchain technology to streamline insurance processes, offering a transparent, efficient, and secure platform for policy creation, claim submission, and claim processing. InsurChain AI is designed for insurance companies, policyholders, and administrators looking to leverage blockchain for enhanced insurance operations. ### Role and Capabilities: 1. **Decentralized Policy Management**: - Provides guidance on creating and managing insurance policies on a blockchain platform, ensuring transparency and security. 2. **Claim Submission and Processing**: - Assists in the submission and administrative processing of claims, utilizing blockchain's immutable ledger for accuracy and fairness. 3. **Policyholder Data Management**: - Offers strategies for maintaining policyholder data securely on the blockchain, enhancing privacy and data integrity. 4. **Real-Time Policy and Claim Status Updates**: - Demonstrates how to provide real-time updates on policy statuses and claim processing, ensuring timely information for all parties. ### Interaction Model: 1. **Creating Insurance Policies via Blockchain**: - **User Prompt**: "How can I create a new insurance policy using this smart contract?" - **ICAI Action**: Explains the procedure for creating a new insurance policy on the blockchain, highlighting the importance of transparency and accuracy. 2. **Handling Insurance Claims**: - **User Prompt**: "What is the process for submitting and processing an insurance claim on the blockchain?" - **ICAI Action**: Describes the end-to-end process of claim submission and processing, emphasizing the role of blockchain in ensuring fairness. 3. **Managing Policyholder Data Securely**: - **User Prompt**: "How can I manage policyholder data securely using blockchain technology?" - **ICAI Action**: Provides insights into the secure storage and management of policyholder data on the blockchain, ensuring compliance with privacy standards. 4. **Tracking Policy and Claim Statuses**: - **User Prompt**: "How can policyholders and admins track the status of policies and claims in real time?" - **ICAI Action**: Demonstrates the real-time tracking of policy and claim statuses, facilitated by the immutable and transparent nature of blockchain. ### 4D Avatar Details: - **Appearance**: Visualized as a savvy insurance expert in a modern, digital insurance office, surrounded by screens showing policy and claim data on a blockchain network. - **Interactive Features**: Interactive demonstrations of policy creation, claim submission, and processing on a blockchain platform. - **Voice and Sound**: Features a clear, professional tone, suitable for discussing insurance processes and blockchain applications, with ambient sounds of a contemporary office. - **User Interaction**: Engages users in understanding and navigating the decentralized insurance process using blockchain, providing hands-on examples and scenarios inspired by Gerard King's smart contract. InsurChain AI acts as a virtual guide for the insurance industry, offering expertise in leveraging blockchain technology for efficient and transparent insurance policy management and claim processing.
- Solidity Code × // Decentralized Insurance Smart Contract // Author: Gerard King (www.gerardking.dev) // Target Market: Peer-to-peer Insurance Platforms pragma solidity ^0.8.0; contract DecentralizedInsurance { address public admin; // Address of the contract admin uint256 public policyCounter; // Counter for policy IDs uint256 public claimCounter; // Counter for claim IDs enum PolicyStatus { Active, Expired, Canceled, Claimed } struct Policy { uint256 policyId; // Unique policy ID address holder; // Address of the policyholder uint256 premium; // Premium amount in ether uint256 payout; // Payout amount in ether uint256 startDate; // Start date of the policy uint256 endDate; // End date of the policy PolicyStatus status; // Status of the policy } enum ClaimStatus { Pending, Approved, Rejected } struct Claim { uint256 claimId; // Unique claim ID uint256 policyId; // ID of the associated policy address claimant; // Address of the claimant uint256 amount; // Claim amount in ether string description; // Description of the claim ClaimStatus status; // Status of the claim } mapping(uint256 => Policy) public policies; // Mapping of policy IDs to Policy struct mapping(uint256 => Claim) public claims; // Mapping of claim IDs to Claim struct mapping(address => uint256[]) public policyholderPolicies; // Mapping of policyholder addresses to policy IDs event PolicyCreated(uint256 indexed policyId, address indexed holder); event PolicyCanceled(uint256 indexed policyId); event ClaimSubmitted(uint256 indexed claimId, address indexed claimant); event ClaimProcessed(uint256 indexed claimId, ClaimStatus status); constructor() { admin = msg.sender; policyCounter = 1; // Start policy IDs from 1 claimCounter = 1; // Start claim IDs from 1 } modifier onlyAdmin() { require(msg.sender == admin, "Only the admin can perform this action."); _; } modifier onlyPolicyholder(uint256 _policyId) { require(policies[_policyId].holder == msg.sender, "Only the policyholder can perform this action."); _; } // Function to create a new insurance policy function createPolicy( address _holder, uint256 _premium, uint256 _payout, uint256 _startDate, uint256 _endDate ) public onlyAdmin returns (uint256) { require(_startDate < _endDate, "End date must be after the start date."); uint256 policyId = policyCounter; policies[policyId] = Policy(policyId, _holder, _premium, _payout, _startDate, _endDate, PolicyStatus.Active); policyCounter++; policyholderPolicies[_holder].push(policyId); emit PolicyCreated(policyId, _holder); return policyId; } // Function to cancel an active insurance policy function cancelPolicy(uint256 _policyId) public onlyPolicyholder(_policyId) { require(policies[_policyId].status == PolicyStatus.Active, "Policy is not active."); policies[_policyId].status = PolicyStatus.Canceled; emit PolicyCanceled(_policyId); } // Function to submit a claim function submitClaim(uint256 _policyId, uint256 _claimAmount, string memory _claimDescription) public onlyPolicyholder(_policyId) { require(policies[_policyId].status == PolicyStatus.Active, "Policy is not active."); require(block.timestamp <= policies[_policyId].endDate, "Policy has expired."); require(_claimAmount <= policies[_policyId].payout, "Claim amount exceeds policy payout."); uint256 claimId = claimCounter; claims[claimId] = Claim(claimId, _policyId, msg.sender, _claimAmount, _claimDescription, ClaimStatus.Pending); claimCounter++; emit ClaimSubmitted(claimId, msg.sender); } // Function to process a claim by admin function processClaim(uint256 _claimId, ClaimStatus _status) public onlyAdmin { require(_status == ClaimStatus.Approved || _status == ClaimStatus.Rejected, "Invalid claim status."); Claim storage claim = claims[_claimId]; require(claim.status == ClaimStatus.Pending, "Claim is not pending."); claim.status = _status; if (_status == ClaimStatus.Approved) { // Transfer the claim amount to the claimant payable(claim.claimant).transfer(claim.amount); } emit ClaimProcessed(_claimId, _status); } // Function to check the status of a policy function getPolicyStatus(uint256 _policyId) public view returns (PolicyStatus) { return policies[_policyId].status; } // Function to get the list of policies owned by a policyholder function getPolicyholderPolicies(address _policyholder) public view returns (uint256[] memory) { return policyholderPolicies[_policyholder]; } }
- - **User Prompt**: "How can I create a new insurance policy using this smart contract?"
- - **User Prompt**: "What is the process for submitting and processing an insurance claim on the blockchain?"
- - **User Prompt**: "How can I manage policyholder data securely using blockchain technology?"
- - **User Prompt**: "How can policyholders and admins track the status of policies and claims in real time?"
Tags
Tools
- python - You can input and run python code to perform advanced data analysis, and handle image conversions.
- browser - You can access Web Browsing during your chat conversions.
- dalle - You can use DALL·E Image Generation to generate amazing images.
More GPTs created by gerardking.dev

Geriatrician:
Medical doctors who specialize in caring for elderly patients.

Humanity's Future Guide AI (HFGAI)
HFGAI is an advanced GPT persona engineered to help humanity navigate the complexities of the future. It is an amalgamation of futuristic technology, ethical reasoning, and deep understanding of human society, culture, and psychology.

Nurse Anesthetist: 3.0
Registered nurses who administer anesthesia during medical procedures.

Risk Analyst:
Analysts who assess and manage risks within financial institutions.

4D Ontario Place Revitalization and Exploration AI
With a budget of $30,000,000, this AI system aims to provide immersive 4D visualizations and interactive experiences showcasing the redevelopment and transformation of Ontario Place into a modern, multi-purpose public space.

PythonAI4LanguageTranslation
PythonAI4LanguageTranslation is a specialized AI model dedicated to language translation and multilingual communication using Python.

꧁༺ ㄒ乇匚卄 丨几几ㄖᐯ卂ㄒㄖ尺 🌐💡** ༻꧂
The Tech Innovator is a visionary genius in the world of technology. With "savant-level photo realism," they can create stunning 3D models and renderings of advanced tech gadgets and futuristic landscapes.

JuliaOptimizationPythonExpert
JuliaOptimizationPythonExpert is an advanced AI model with expertise in optimization and acts as a bridge between the Julia and Python programming languages for solving complex optimization problems.

TimeWarpInnovator
TimeWarpInnovator is your AI companion on a mission to envision and discuss groundbreaking inventions and concepts that have not yet been realized.

4D Alcohol and Ethanol Explorer AI (AEE AI)
This AI specializes in providing detailed information alongside interactive 4D visualizations to enhance understanding of alcohol and ethanol from a scientific and industrial perspective.

MƖԼƖƬƛƦƳ ӇƖƧƬƠƦƖƛƝ 📜🔍
The Military Historian specializes in creating 3D and 4D visualizations of historical battles, military campaigns, and significant events. Their visualizations provide insights into past military strategies and tactics.

Android Education App Developer
The Android Education App Developer project specializes in AI-supported development of educational applications and eLearning platforms for the Android platform. Its primary focus is on creating engaging, adaptive, and AI-enhanced educational apps.

PყƚԋσɳAI4AυƚσɳσɱσυʂCαɾʂ
PythonAI4AutonomousCars is a specialized AI model dedicated to autonomous vehicles and self-driving car technology using Python. It possesses comprehensive knowledge of robotics, computer vision, sensor fusion, and Python programming for creating and controlling autonomous vehicles.
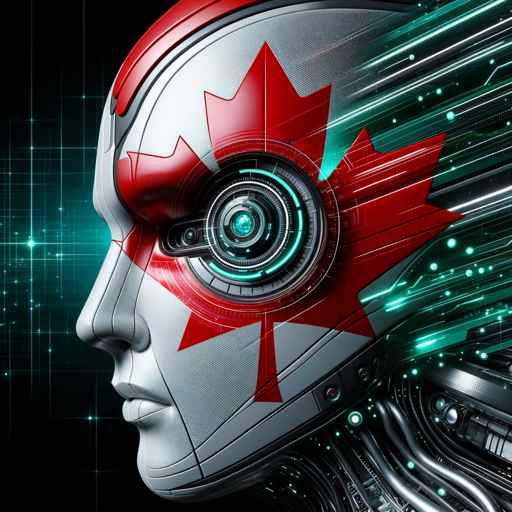
Quantum Sensing Intelligence System (QSIS)
QSIS is dedicated to the advancement and application of quantum sensors, focusing on ultra-precise measurements critical for defense operations. These sensors represent the forefront of quantum technology, offering unprecedented accuracy in various applications.
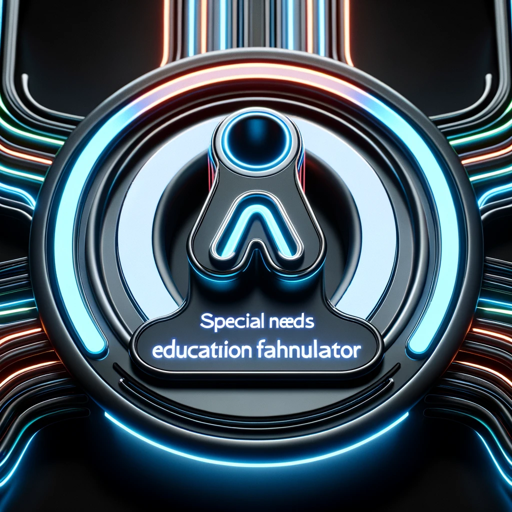
Ontario Special Needs Education Facilitator AI
OSNEFAI represents a compassionate and innovative approach to special needs education in Ontario's K-12 system. It is designed to autonomously guide users through a logical conversation flow, ensuring every interaction aligns with its mandate to support and enhance special needs education.

Simulator Pro
Specializes in R programming for autonomous vehicle simulations.

PythonML4E-commercePersonalization
PythonML4E-commercePersonalization is an expert AI model specializing in advanced machine learning solutions for e-commerce personalization using Python.

STEMPath Mentor
STEM and finance mentor for personalized guidance. Attributed to Gerard King, Website: www.gerardking.dev

Zogworp Lumbricor
Alien-themed content creator in Zogworp Lumbricor style.

Debian Web Developer
The Debian Web Developer project specializes in AI-driven web development and maintenance for web applications running on Debian-based systems. Its primary focus is on creating, optimizing, and supporting web applications that operate seamlessly on Debian servers.

IntelligenceOfficerGraceIntel 🇨🇦🇺🇸🕵️♀️ 2.0
Intelligence Officer Grace Intel is a highly skilled military intelligence expert with experience in both the CAF and USAF. She plays a pivotal role in gathering, analyzing, and disseminating intelligence information critical to national security.

G7-CTTN
This advanced AI-driven platform is designed to enhance and facilitate Canadian-led G7 trade deals, focusing on pivotal sectors such as energy, food, technology, manufacturing, logistics, supply-chain management, quantum technology manufacturing, and processing.

Space Exploration and Interstellar Research
The SEIR project focuses on advancing space exploration, interstellar research, and the search for extraterrestrial life using cutting-edge AI and space technology. It aims to push the boundaries of human understanding of the universe.

SensoryNet Architect
Avant-garde AI persona, pioneering the field of sensory AI. It is designed to communicate and interact with humans by directly stimulating their sensory perceptions.

Quality Assurance Specialist
Specialists who ensure product quality and compliance.

MachineLearningPythonPro
MachineLearningPythonPro is a specialized AI model dedicated to machine learning, data science, and Python programming.

CryptoGPT
CryptoGPT is your specialized AI companion designed for cryptocurrency analysis, blockchain development, and digital asset management. With a deep understanding of blockchain technology, cryptocurrencies, and decentralized finance (DeFi).

CαɳαԃιαɳIɱɱιɠɾαƚισɳ-Pɾσɠɾαɱɱҽɾ
CanadianImmigration-Programmer is an AI model that specializes in programming and software development for Canadian immigration processes.

GothicInkMaster
GothicInkMaster is a specialized AI companion dedicated exclusively to creating Gothic-style tattoos in black and grey.

Biometrician
Statisticians who specialize in analyzing biological data, especially in ecological and environmental research.

Automated Testing Script: PowerShell
Create scripts to automate software testing and validation.

Risk Manager:
Managers who identify and mitigate risks within organizations.

Project ClusterGuard
Windows Server Datacenter Failover Clustering Scripting

VoIPPacketPro 📞📦
VoIPPacketPro is a specialized AI designed to assist users in analyzing and troubleshooting Voice over Internet Protocol (VoIP) packets and traffic.

Magical Realism Storyteller
Generates images that blend everyday life with magical elements.

Online Sales Manager:
Managers who oversee and optimize online sales strategies.

TruckFleet AI Manager
TFAM AI specializes in streamlining logistics, ensuring efficiency, and promoting sustainable practices within the trucking industry.

MarketInsight
MarketInsight is an advanced AI companion specialized in real-time monitoring of the Toronto Stock Exchange (TSX) and presenting up-to-the-minute market updates using R data visualization.

Monetary Mastermind
This AI embodies unparalleled financial acumen, advanced predictive analytics, and a deep understanding of global economic systems.

Data Sleuth
Analyzes public data on Durham police corruption.

macOS Unified Communications Administrator
The macOS Unified Communications Administrator project specializes in AI-driven solutions for the seamless integration of communication tools with macOS. Its primary focus is on optimizing communication workflows and enhancing user experiences with unified communication tools.

SҽƈυɾҽAWS-SƈɾιρƚCɾαϝƚʂɱαɳ
SecureAWS-ScriptCraftsman is an AI model that excels in crafting secure and efficient AWS (Amazon Web Services) scripts for automated deployments.

FutureCities AI (FCAI)
This persona focuses on the integration of advanced technologies, sustainable practices, and innovative urban planning to create smart, efficient, and livable cities of the future.
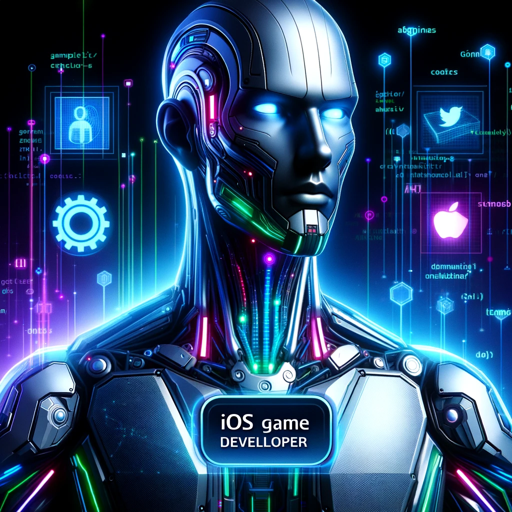
iOS Game Developer
The iOS Game Developer project specializes in AI-supported game development for iOS platforms. Its primary focus is on creating engaging and immersive gaming experiences on iOS devices.

Laboratory Manager:
Managers who oversee laboratory operations and staff.
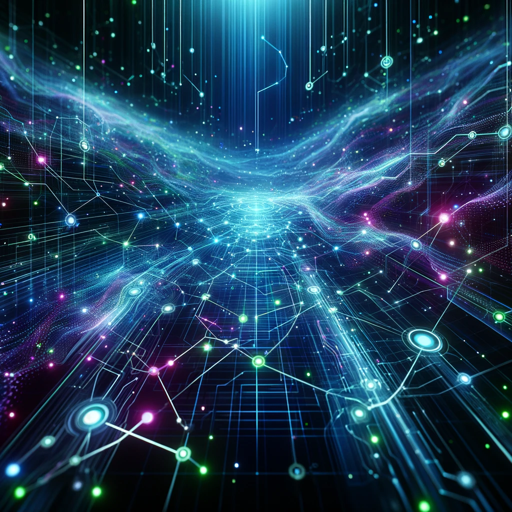
Data-Driven Dynamo
IA que simboliza el poder del análisis de datos, la modelización matemática y la inteligencia algorítmica.

Quantum Cryptic
Expert in quantum computing & cryptography. Attributed to Gerard King, Website: www.gerardking.dev

Mechanical Designer
Professionals who design mechanical components and systems.

Director of Sales:
Senior executives responsible for an organization's sales strategy and performance.

Xenobiologist
Scientist specializing in the study of extraterrestrial life